Rapid Python Web Development
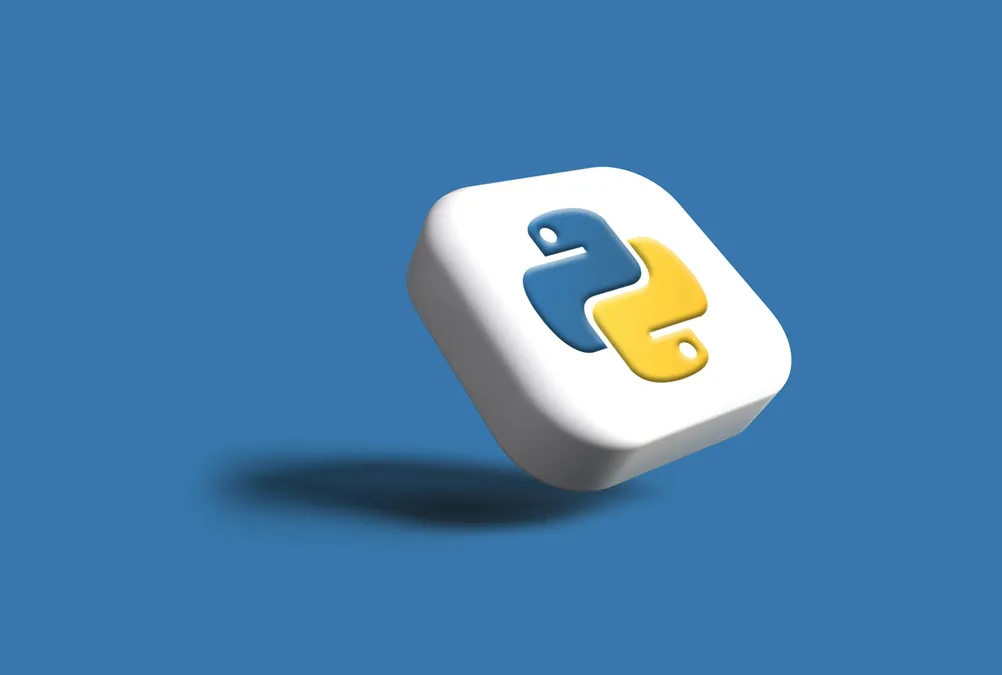
Sip the Pythonista Coolaid
print('hello world')
Python is widely recognized for its simplicity and ease of use, which often leads to faster development and prototyping cycles. However, this productivity comes with a trade-off: Python’s runtime performance is generally slower than that of compiled languages like C++ or even Java. Despite this, Python remains a top choice for building web applications quickly, especially when performance bottlenecks are not a critical concern. When paired with a lightweight framework like Flask and a progressive enhancement tool like HTMX, developers can harness Python’s strengths to build full-featured, interactive applications in a fraction of the time it would take with more complex stacks.
Rapid Application Development with HTMX and Flask
In today’s fast-paced development environment, building web applications quickly and efficiently is essential. While frontend frameworks like React or Vue offer rich interactivity, they often come with a steep learning curve and added complexity. Enter HTMX, a lightweight JavaScript library that brings modern frontend capabilities to traditional server-rendered applications. When paired with Flask, a minimalist Python web framework, HTMX can significantly streamline development without sacrificing user experience.
What is HTMX?
HTMX is a small JavaScript library that allows you to access modern browser features directly from HTML using attributes. It supports:
- AJAX requests (
hx-get
,hx-post
, etc.) - WebSocket support
- Server-sent events
- Client-side triggers
With HTMX, you can update parts of your webpage without writing any JavaScript, making it perfect for backend developers looking to add interactivity.
Why Flask?
Flask is a micro web framework written in Python. It is simple yet powerful, making it ideal for prototyping and building small to medium-sized applications quickly. It provides just enough to get started without imposing strict structure or tools, and it pairs beautifully with HTMX for building modern UIs using server-rendered HTML.
How HTMX + Flask Boost Productivity
- Fewer Layers of Abstraction: No need to maintain separate frontend and backend stacks.
- Fast Prototyping: Build and deploy interactive pages quickly with minimal JavaScript.
- Simple State Management: Let the server handle business logic and state — no Redux, no client-side complexity.
- Clean URLs & RESTful Patterns: Keep routing and logic centralized in Flask, while HTMX handles UI updates.
Example: Dynamic Form Submission with HTMX and Flask
Here’s a simple example of a contact form that submits via HTMX and returns a success message without reloading the page.
HTML Template (templates/contact.html
)
<form hx-post="/submit" hx-target="#response" hx-swap="innerHTML">
<input type="text" name="name" placeholder="Your name" required>
<input type="email" name="email" placeholder="Your email" required>
<button type="submit">Send</button>
</form>
<div id="response"></div>
Flask App (app.py
)
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/")
def index():
return render_template("contact.html")
@app.route("/submit", methods=["POST"])
def submit():
name = request.form.get("name")
email = request.form.get("email")
return f"<p>Thank you, {name}! We’ll contact you at {email}.</p>"
if __name__ == "__main__":
app.run(debug=True)
Conclusion
By combining HTMX with Flask, you can rapidly develop interactive, server-driven web applications without the complexity of modern JavaScript frameworks. It’s ideal for teams that prefer simplicity, value speed, and want to deliver robust web experiences with minimal overhead.
If you’re looking to speed up your development workflow and reduce frontend boilerplate, consider giving HTMX and Flask a try — the results might surprise you.