How to make PHP great
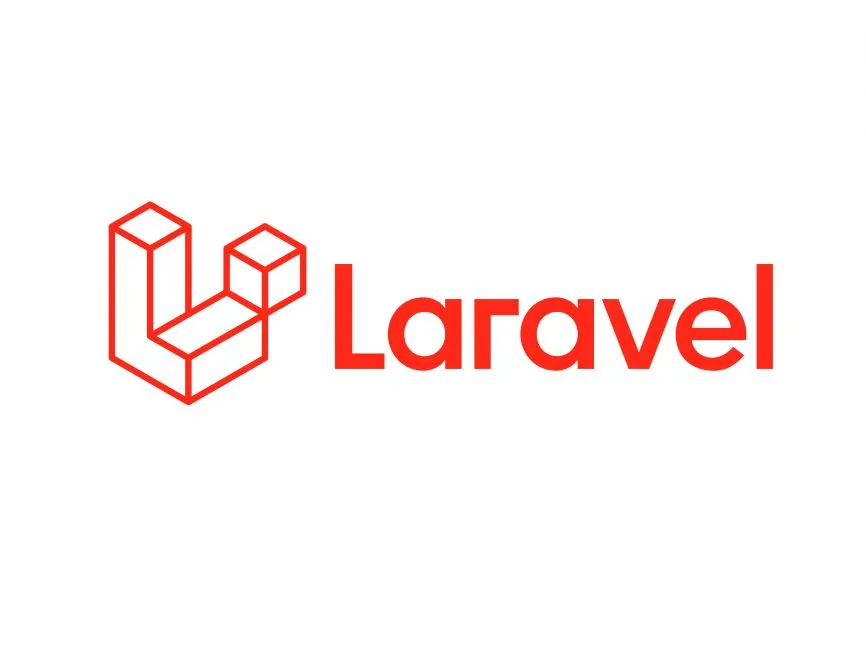
The Modern Way to Do PHP: Laravel in 2025
PHP has come a long way from the days of spaghetti code and WordPress-only use cases. If you’ve written off PHP in the past, now is the time to take another look — especially if you’re working on modern web applications. Laravel, in particular, has redefined the developer experience, combining elegant syntax with a powerful ecosystem that’s hard to beat.
Whether you’re building a full-stack app or just need a clean API layer, Laravel gives you the tools to move fast, stay organized, and deliver maintainable code — all without sacrificing performance or structure.
🚀 The Laravel Ecosystem
One of Laravel’s biggest strengths is its tightly integrated ecosystem. Out of the box, you get:
- Laravel Breeze / Jetstream: Prebuilt auth scaffolding
- Eloquent: Laravel’s active record ORM
- Laravel Livewire: Full interactivity without writing JavaScript
- Laravel Nova: Admin panels made easy
- Laravel Forge / Vapor: Deployment and serverless hosting
- Laravel Sanctum / Passport: First-party API authentication tools
Everything just works — and that cohesion means less time setting up and more time building.
🌲 TALL Stack: Tailwind, Alpine.js, Laravel, Livewire
The TALL stack is Laravel’s answer to full-stack JavaScript frameworks — but without writing much JavaScript at all.
- TailwindCSS for styling
- Alpine.js for tiny bits of interactivity
- Laravel as the backend
- Livewire to connect your frontend and backend
Livewire in particular is game-changing: it allows you to write dynamic components using only PHP and Blade templates, with no Vue or React setup required.
<!-- resources/views/components/counter.blade.php -->
<div>
<button wire:click="increment">+</button>
<span>{{ $count }}</span>
</div>
It feels like magic — especially when you’re used to wiring up client-side state manually.
🤔 But About ORMs…
My only real issue with Laravel is something that’s more of a personal preference: I generally hate ORMs.
What’s an ORM?
ORM stands for Object-Relational Mapping — a layer that lets you interact with your database using objects instead of raw SQL. Laravel’s ORM is called Eloquent, and it looks like this:
$users = User::where('active', 1)->orderBy('name')->get();
While this is clean and expressive, ORMs often abstract away too much. They can lead to inefficient queries, especially if you don’t understand the underlying SQL. I tend to prefer writing raw or parameterized queries directly, or using a lightweight query builder like Laravel’s own DB
facade:
$users = DB::table('users')->where('active', 1)->orderBy('name')->get();
That said, for teams that value readability and convention, Eloquent is very productive — just keep an eye on query performance and N+1 problems.
📦 Other Laravel-Led Modern Stacks
Beyond TALL, Laravel works well with:
- Inertia.js: Let’s you build SPAs using Vue, React, or Svelte, but keeps routing and server rendering in Laravel.
- Statamic / Filament / Laravel Nova: Powerful CMS or admin interfaces with Laravel under the hood.
- Laravel Octane: Supercharges performance by using Swoole or RoadRunner for persistent workers and faster request handling.
No matter what your preferred frontend or deployment style, there’s probably a Laravel-powered option for it.
🧑💻 Final Thoughts
Laravel isn’t just “the modern way to do PHP” — it’s one of the most productive and elegant ways to build web applications, period. The syntax is clean, the documentation is top-tier, and the ecosystem is deep. Even if you’re not in love with Eloquent or ORMs in general, Laravel’s flexibility means you can still use raw SQL, custom query builders, or integrate external services easily.
If it’s been a while since you touched PHP, give Laravel another look. It might just surprise you.
Resources
- laravel.com
- laracasts.com — free and premium screencasts
- TALL stack docs
- Laravel Forge
- Laravel Nova